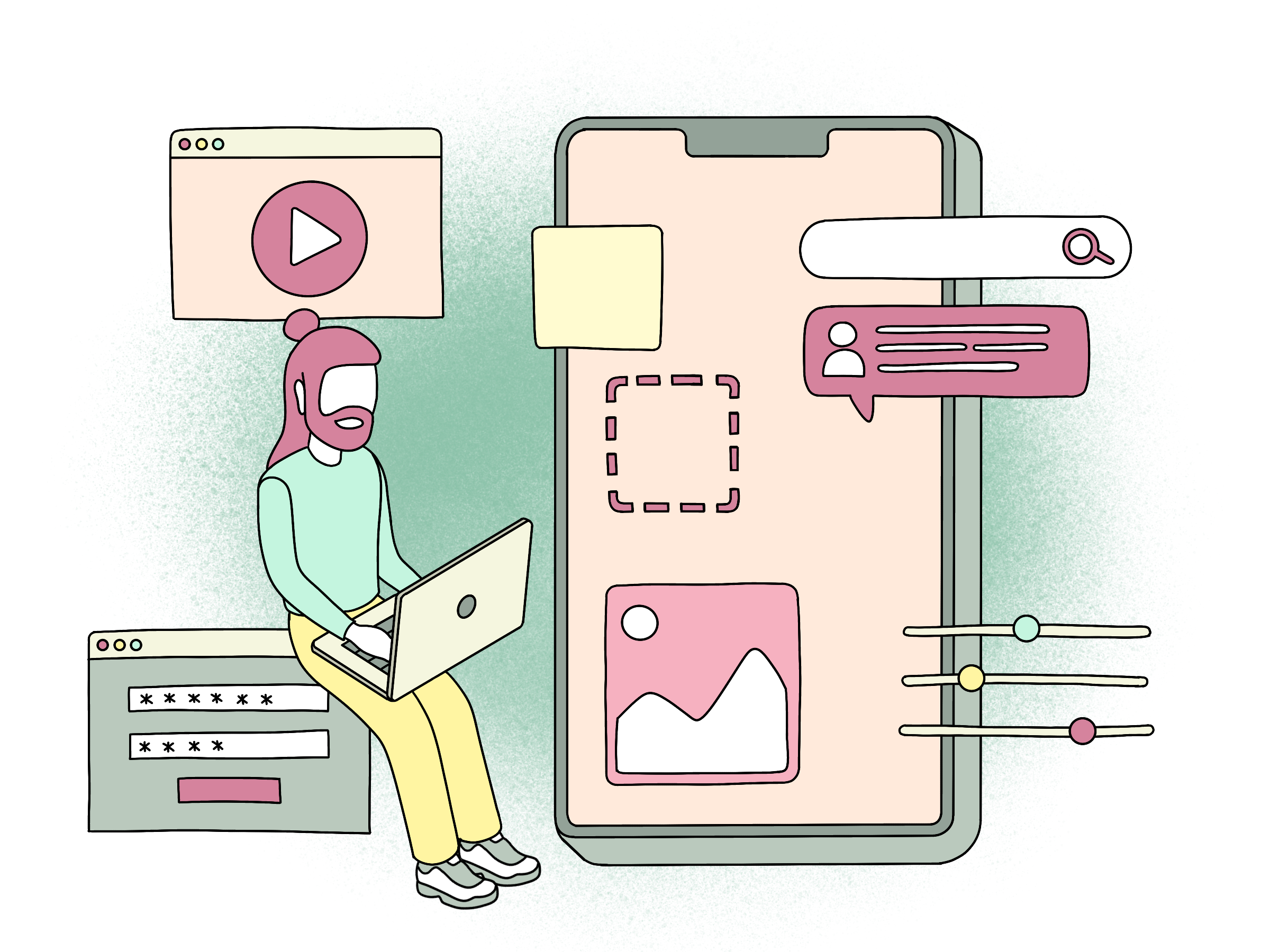
Responsive images in React with srcset
Images are essential in web and, together with videos, they make up for the most bytes downloaded in most websites. How do we serve the correct images to our users in React?
Why is this important?
Images are essential in web and, together with videos, they make up for the most bytes downloaded in most websites. To illustrate: here is how much of the downloaded bytes are images for a few popular sites
telegraph.co.uk: 42%
youtube.com: 27%
amazon.co.uk: 95%
netflix.com: 65%
stackoverflow.com: 38%
So, since you also most likely have a lot of image on your site, two important questions needs to be answered:
- How do I ensure high quality images for my users?
- How do I ensure that they don’t download unnecessary big images?
You cannot just serve huge images to everyone, it will affect the performance for users on a medium/slow connection. Those users will most likely have significant spend less time on your page, and conversion rate will be lower if you are running an ecommerce. What’s the solution?
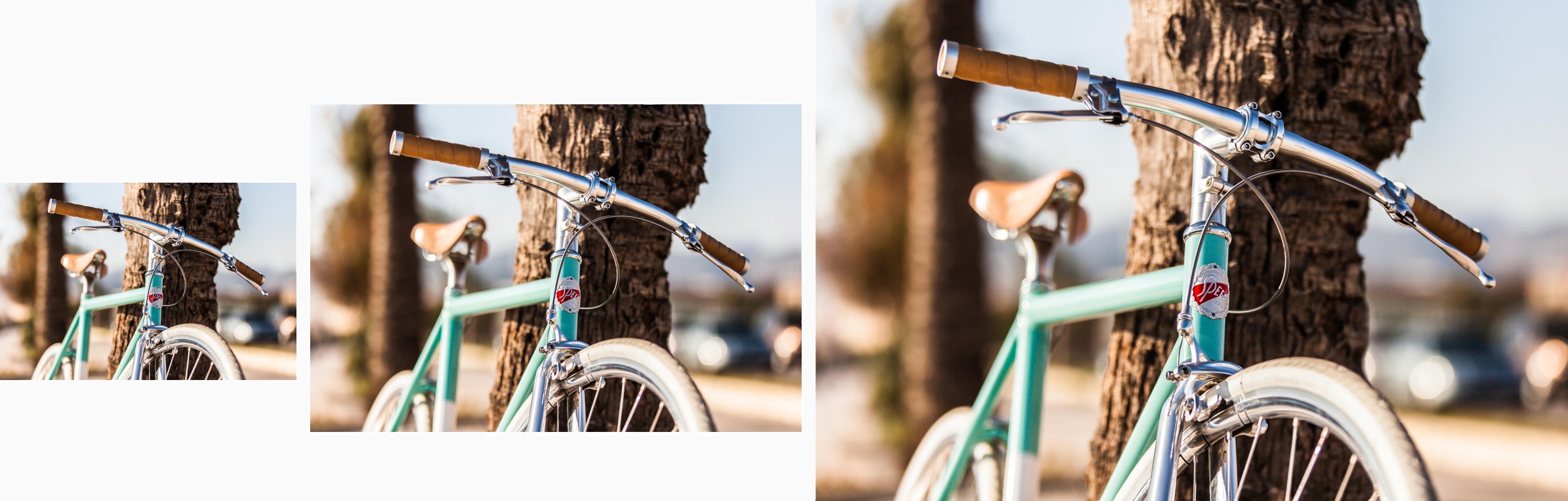
Solutions
Historically there have been many attempts to solve this. Most of these attempts have been using Javascript, where the correct image to serve was determined by checking the device screen width along with the device pixel ratio after the page is loaded. With this you can make a fair assumption on which image to serve. There are however two major problems with this:
- The calculation happens after the page is loaded. What would you show before that?
- What happens when the browser landscape changes, and your algorithm is out of date? And how do you know when it is out of date?
Enter React srcset
A while ago, the srcset attribute was introduced on <img> tags. This is a powerful attribute which enables the browser to determine which image to serve the user! No javascript, no waiting for page to load!
It can be implemented in React in like so:
const small = '300.jpg';
const medium = '768.jpg';
const large = '1280.jpg';
const ResponsiveImage = () => (
<img src={small} srcSet={`${small} 300w, ${medium} 768w, ${large} 1280w`} />
);
Out whole site is implemented with this, but if you want to try for yourself have a look at this pen:
https://codepen.io/snowballdigital/pen/KQGvYJ?editors=0010
You can also check out the React Image Srcset package by Crystallize.
If you are building a React ecommerce solution you should check out Crystallize which has built in support for responsive images. Great for frontend performance and ecommerce seo.
Conclusion
Srcset has gained incredible support in the browser landscape, and there is no reason for not using it today! If you want even more fine-grained control, check out the sizes attribute and the source element!