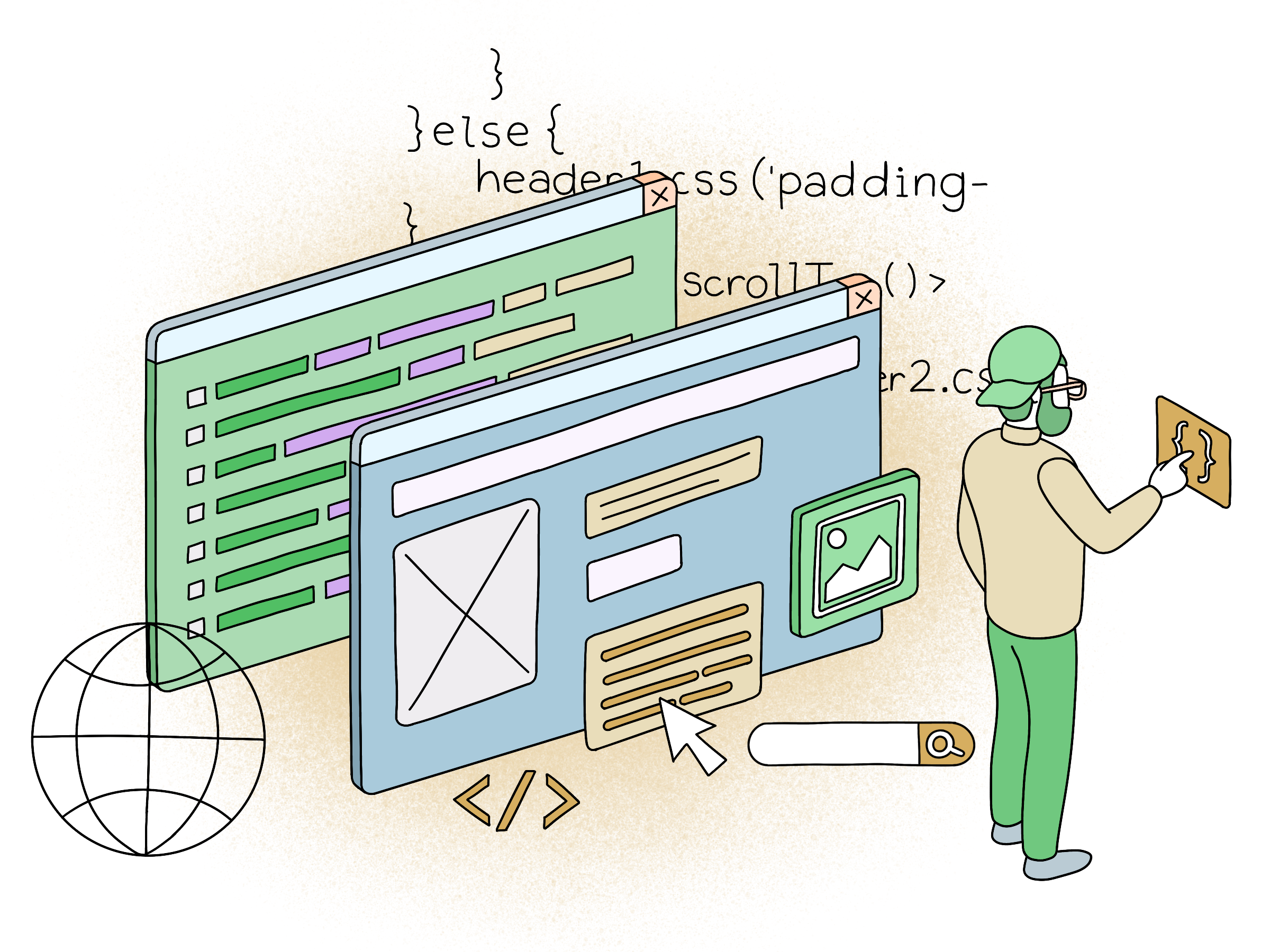
Switch between front and back Camera in React Native
So let’s extend our camera app even further, in this nifty little blog post we will implement a button to switch between the front and back camera on your phone
If you have not read our two previous blog posts on how to get started on an React Native Camera App I suggest you take a look at Capturing Pictures in React Native.
At the moment our camera component is stateless, so first off we need to add a constructor and a couple of states.
constructor(props) {
super(props);
this.state = {
cameraType : 'back',
mirrorMode : false
}
}
Now that we a couple of states we should use these to define our initial camera options. As you can see I also added a mirrormode state, this is because we need to return a mirrored image on capture for all those selfies.
In the camera declaration add these two lines:
type={this.state.cameraType}
mirrorImage={this.state.mirrorMode}
Easy enough huh? Lets add a function to switch between our two cameras.
changeCameraType() {
if (this.state.cameraType === 'back') {
this.setState({
cameraType: 'front',
mirror: true
});
} else {
this.setState({
cameraType: 'back',
mirror: false
});
}
}
Next we need to add something amazing to click on, but let’s be lazy and still use a text block as button for now, we will focus on making this app sparkle at a later stage.
Add this line somewhere inside you camera:
<Text style={styles.capture} onPress={this.changeCameraType.bind(this)}>
[SWITCH O ROONEY]
</Text>;
And thats it! Get on rocking with those selfies!
The screenshot below shows how your camera application should look now
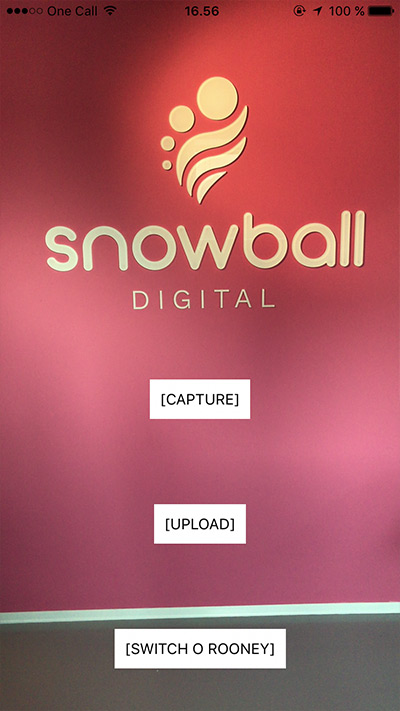
And for all of you TLDR people, here is the full view so you can copy paste it to your project.
import React, { Component } from 'react';
import { AppRegistry, StyleSheet, Text, Dimensions, View } from 'react-native';
import Camera from 'react-native-camera';
const PicturePath = '';
export default class MyCamera extends Component {
constructor(props) {
super(props);
this.state = {
cameraType: 'back',
mirrorMode: false
};
}
render() {
return (
<View style={styles.container}>
<Camera
ref={cam => {
this.camera = cam;
}}
style={styles.preview}
aspect={Camera.constants.Aspect.fill}
captureTarget={Camera.constants.CaptureTarget.disk}
type={this.state.cameraType}
mirrorImage={this.state.mirrorMode}
>
<Text style={styles.capture} onPress={this.takePicture.bind(this)}>
[CAPTURE]
</Text>
<Text style={styles.capture} onPress={this.storePicture.bind(this)}>
[UPLOAD]
</Text>
<Text
style={styles.capture}
onPress={this.changeCameraType.bind(this)}
>
[SWITCH O ROONEY]
</Text>
</Camera>
</View>
);
}
changeCameraType() {
if (this.state.cameraType === 'back') {
this.setState({
cameraType: 'front',
mirrorMode: true
});
} else {
this.setState({
cameraType: 'back',
mirrorMode: false
});
}
}
storePicture() {
if (PicturePath) {
// Create the form data object
var data = new FormData();
data.append('picture', {
uri: PicturePath,
name: 'selfie.jpg',
type: 'image/jpg'
});
// Create the config object for the POST
// You typically have an OAuth2 token that you use for authentication
const config = {
method: 'POST',
headers: {
Accept: 'application/json',
'Content-Type': 'multipart/form-data;',
Authorization: 'Bearer ' + 'SECRET_OAUTH2_TOKEN_IF_AUTH'
},
body: data
};
fetch('https://postman-echo.com/post', config)
.then(responseData => {
// Log the response form the server
// Here we get what we sent to Postman back
console.log(responseData);
})
.catch(err => {
console.log(err);
});
}
}
takePicture() {
this.camera
.capture()
.then(data => {
console.log(data);
PicturePath = data.path;
})
.catch(err => console.error(err));
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF'
},
preview: {
flex: 1,
justifyContent: 'flex-end',
alignItems: 'center',
height: Dimensions.get('window').height,
width: Dimensions.get('window').width
},
capture: {
flex: 0,
backgroundColor: '#fff',
borderRadius: 5,
color: '#000',
padding: 10,
margin: 40
}
});
AppRegistry.registerComponent('MyCamera', () => MyCamera);