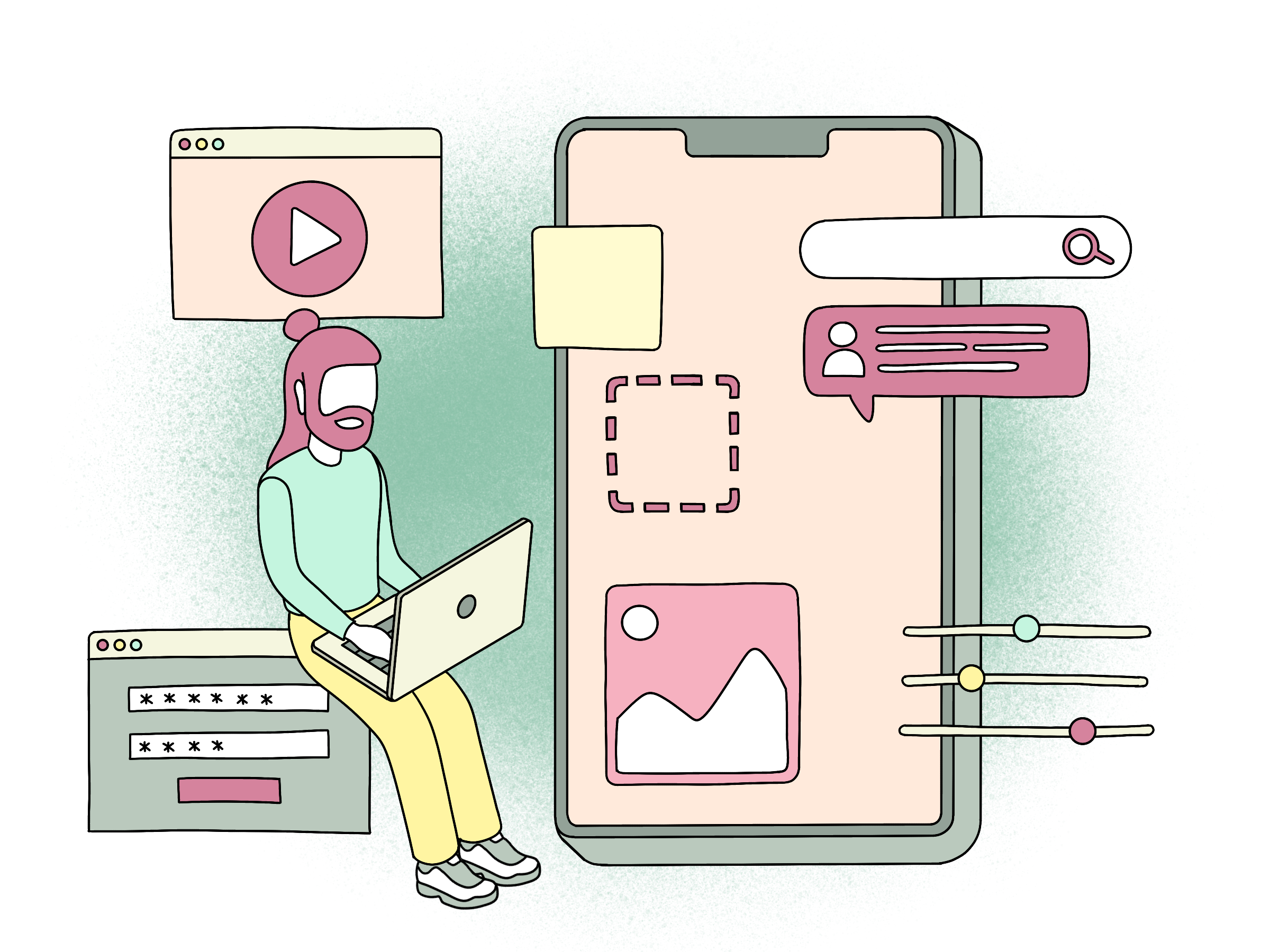
Uploading Images in React Native
When you are working with React Native and images you are likely to want to upload them somewhere. Here is how I did this.
This is a continuation of my previous blog post on Capturing Images in React Native.
The first thing is to create a variable where you can keep the path for your image. I just created a variable called PicturePath for simplicity.
Then you have to add the captureTarget to your camera component. I chose to create a new button, upload, for the action of pushing my image to a server.
captureTarget={Camera.constants.CaptureTarget.disk}><Text style={styles.capture} onPress={this.storePicture.bind(this)}>[UPLOAD]</Text>
Upload picture from iPhone to server
Before we can upload a picture we have to capture it. This is done in the takePicture function. From my previous example I just modified this to store the PicturePath upon successful capture of the image from the camera.
takePicture() {
this.camera.capture().then(data => {
console.log(data);
PicturePath = data.path;
}).catch(err => console.error(err));
}
The function storePicture does a couple of things. First it checks if the PicturePath is set. Meaning that if does not try to upload if we have not yet taken a picture.
We then create the form data object. This actually handles the Base64 encoding for our upload.
The POST method is then configured. Here you have to set the multipart form-data to indicate that you are sending an actual form with a file. I also added an Authorization header, this is typical if you are using OAuth2 authorization with the server.
To do the actual upload we are using the fetch function. This is quite useful to send GET, PUT, POST, PATCH or DELETE requests over REST. In this case we add the endpoint URL parameter and our config we already created.
When we get a successful response from the server we are just doing a console log here. This is where you would check server status and message the user of a successful upload. But I am keeping this example lean.
storePicture() {
console.log(PicturePath);
if (PicturePath) {
// Create the form data object
var data = new FormData();
data.append('picture', {
uri: PicturePath,
name: 'selfie.jpg',
type: 'image/jpg'
});
// Create the config object for the POST
// You typically have an OAuth2 token that you use for authentication
const config = {
method: 'POST',
headers: {
Accept: 'application/json',
'Content-Type': 'multipart/form-data;',
Authorization: 'Bearer ' + 'SECRET_OAUTH2_TOKEN_IF_AUTH'
},
body: data
};
fetch('https://postman-echo.com/post', config).then(responseData => {
// Log the response form the server
// Here we get what we sent to Postman back
console.log(responseData);
}).catch(err => { console.log(err); });
}
}
A tip when developing a REST client in React Native, or other client languages, is to use Postman Echo to test your initial endpoints. It is useful
Below is a screenshot of what the camera App looks like at this point. Not fancy, but still. It can take a picture and upload it to a server.
You can check out the complete code on our MyCamera on GitHub.
GraphQL based backend for React and React Native?
Crysatllize is a back-end for rich product information and e-commerce built on GraphQL. Ideal for React and React Native e-commerce Apps.
Check out Headless Commerce with Crystallize.